Python Metaprogramming and Decorators: Enhancing Code Dynamics
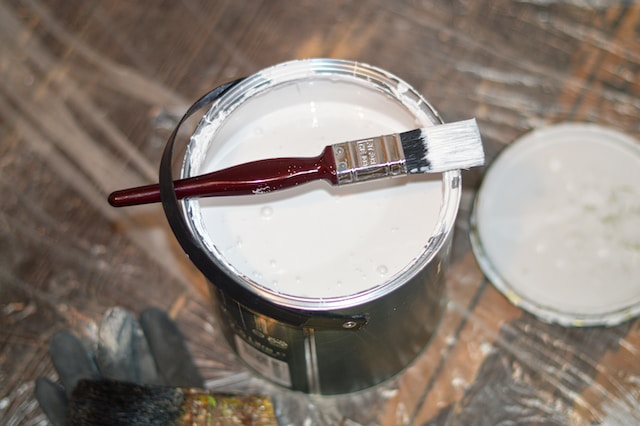
Python, being a dynamic language, provides robust tools for metaprogramming – the art of writing code that manipulates itself. One such metaprogramming tool that stands out is the 'decorator'. They not only improve code readability but also introduce added functionalities seamlessly.
A Glimpse into Metaprogramming
Metaprogramming is the practice of writing programs that can modify, generate, or manipulate other programs (or themselves). In Python, this often translates to altering classes, functions, or modules at runtime.
Examples include: - Dynamic attribute addition to objects. - Modifying classes with class decorators. - Wrapping or modifying functions with decorators.
Deciphering Decorators
Decorators, in essence, are design patterns in Python, allowing you to add new functionalities to existing objects without altering their structure. They're often prefixed with @
and placed above functions or methods.
A Basic Decorator:
def simple_decorator(func):
def wrapper():
print("Before the function call")
func()
print("After the function call")
return wrapper
@simple_decorator
def say_hello():
print("Hello!")
say_hello()
Executing say_hello()
would print:
Before the function call
Hello!
After the function call
The @simple_decorator
is a syntactic sugar for: say_hello = simple_decorator(say_hello)
.
Practical Applications of Decorators
-
Logging: Track metadata about function execution times, arguments, or results.
-
Authorization: Check if someone's authorized to use an API endpoint, a method, etc.
-
Enrichment: Modify the results or inputs of functions, e.g., changing the datatype, adding default values, or computing additional data.
-
Memoization: Store results of expensive function calls and return the cached result when the same inputs occur again.
Crafting Custom Decorators
To elevate code legibility and upkeep:
-
With Arguments:
Decorators can also accept arguments, allowing you to further customize behavior. For instance, a decorator to repeat the execution of a function
n
times:
def repeat(n=2):
def decorator(func):
def wrapper(*args, **kwargs):
for _ in range(n):
result = func(*args, **kwargs)
return result
return wrapper
return decorator
@repeat(n=3)
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
This prints "Hello, Alice!" thrice.
-
Class Decorators:
For enhancing or modifying classes:
def readonly_properties(*attrs):
def class_decorator(cls):
for attr in attrs:
prop = property(cls.__dict__[attr])
setattr(cls, attr, prop)
return cls
return class_decorator
@readonly_properties('name')
class Person:
def __init__(self, name):
self._name = name
def name(self):
return self._name
p = Person("John")
# p.name = "Doe" # This will raise an error
The readonly_properties
decorator makes class properties read-only, preventing unintended changes.
Conclusion
Decorators are Python's gems, allowing for code augmentation without direct modifications. They embody the essence of metaprogramming, offering the ability to enhance, control, and extend functionalities. By integrating decorators into your toolkit, you empower your codebase, ensuring it remains DRY (Don't Repeat Yourself), readable, and maintainable.