Designing a Notification Service using Python
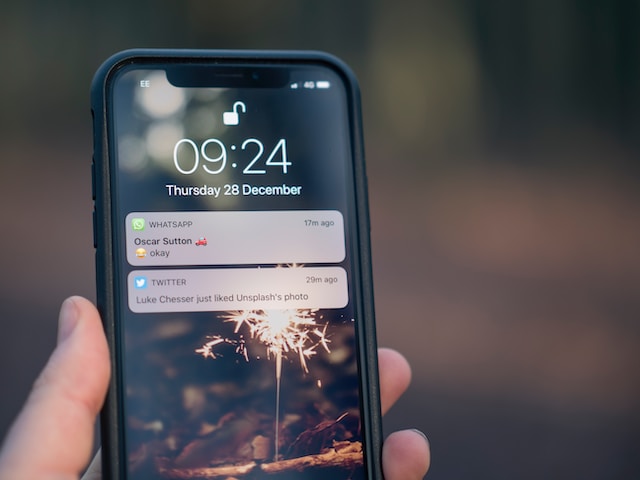
In today's digital landscape, a flexible notification service that can send messages via different backend providers is invaluable. By leveraging platforms such as SendGrid for emails, Twilio for SMS, Telegram for messaging, and OneSignal for push notifications, businesses can reach users across a spectrum of communication channels.
This guide illustrates how to create a Python-based notification service that seamlessly integrates with multiple providers.
Requirements:
- Python 3.x
- SDKs and third-party libraries:
requests
,sendgrid
,twilio
, etc.
Designing the Service
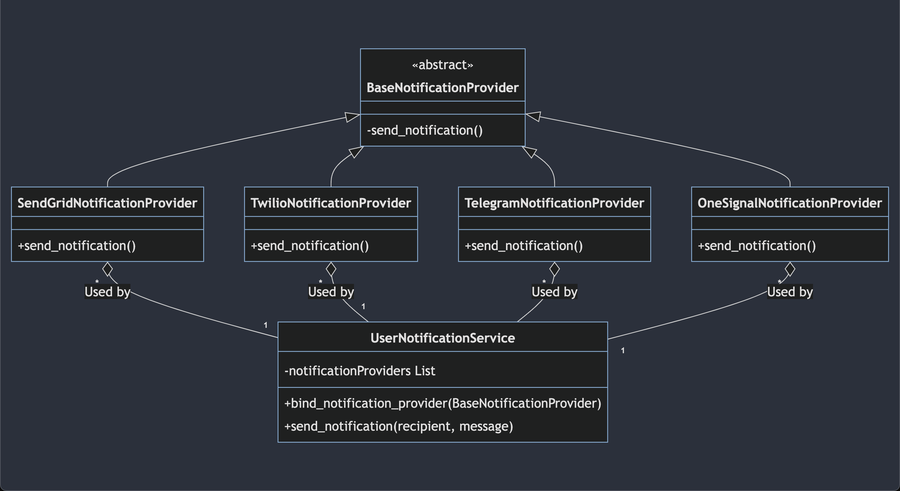
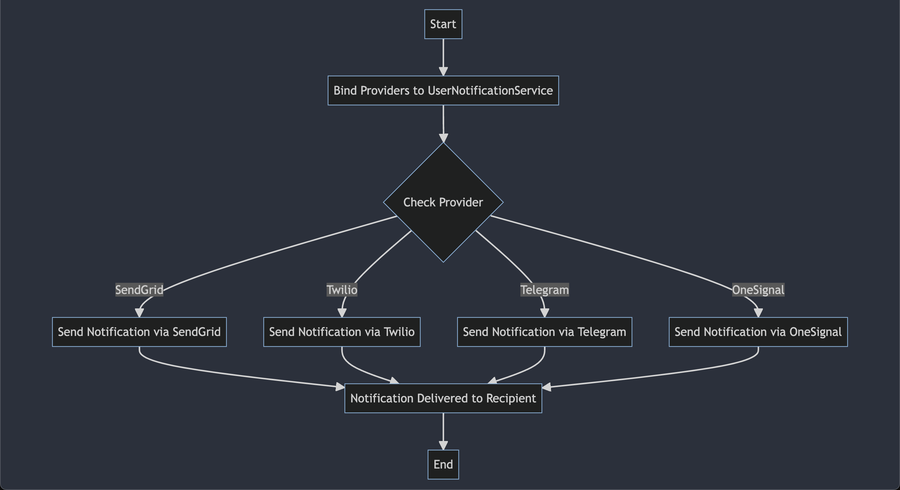
Base Notification Provider Class
At the core is an abstract class that offers a basic framework for individual provider integrations.
class BaseNotificationProvider:
def send_notification(self, recipient, message):
raise NotImplementedError("This method should be implemented by the child class.")
Provider-Specific Classes
Concrete classes for each backend provider will inherit from the base class and implement the send_notification method.
SendGrid
from sendgrid import SendGridAPIClient
from sendgrid.helpers.mail import Mail
class SendGridNotificationProvider(BaseNotificationProvider):
def __init__(self, api_key):
self.client = SendGridAPIClient(api_key)
def send_notification(self, recipient, message):
mail = Mail(from_email='your_email@example.com', to_emails=recipient, subject='Notification',
html_content=message)
self.client.send(mail)
Twilio
from twilio.rest import Client
class TwilioNotificationProvider(BaseNotificationProvider):
def __init__(self, account_sid, auth_token):
self.client = Client(account_sid, auth_token)
def send_notification(self, recipient, message):
self.client.messages.create(body=message, to=recipient, from_="Your_Twilio_Number")
Telegram
To interact with Telegram's Bot API, you can use the python-telegram-bot package.
import telegram
class TelegramNotificationProvider(BaseNotificationProvider):
def __init__(self, bot_token):
self.bot = telegram.Bot(token=bot_token)
def send_notification(self, chat_id, message):
self.bot.send_message(chat_id=chat_id, text=message)
OneSignal
import requests
class OneSignalNotificationProvider(BaseNotificationProvider):
def __init__(self, app_id, api_key):
self.app_id = app_id
self.api_key = api_key
def send_notification(self, recipient, message):
headers = {
"Authorization": f"Basic {self.api_key}",
"Content-Type": "application/json"
}
payload = {
"app_id": self.app_id,
"contents": {"en": message},
"include_player_ids": [recipient]
}
requests.post("https://onesignal.com/api/v1/notifications", headers=headers, json=payload)
Managing Notifications Across Providers
For users who have bound multiple providers, the service must send notifications across all these channels.
class UserNotificationService:
def __init__(self):
self.notificationProviders = []
def bind_notification_provider(self, *providers):
"""Bind a new notification provider to the user."""
self.notificationProviders.extend(list(providers))
def send_notification(self, recipient, message):
for provider in self.notificationProviders:
provider.send_notification(recipient, message)
Usage
user_service = UserNotificationService()
# Bind SendGrid, Twilio, Telegram, and OneSignal for a user
user_service.bind_notification_provider(
SendGridNotificationProvider('YOUR_SENDGRID_API_KEY')),
TwilioNotificationProvider('YOUR_TWILIO_SID', 'YOUR_TWILIO_TOKEN')),
TelegramNotificationProvider('YOUR_TELEGRAM_BOT_TOKEN')),
OneSignalNotificationProvider('YOUR_ONESIGNAL_APP_ID', 'YOUR_ONESIGNAL_API_KEY'),
)
# Send a notification
user_service.send_notification('recipient@example.com', 'Hello from multi-provider notification service!')
This service will dispatch the given message via all bound providers for that user.
Conclusion
Creating a multi-provider notification service in Python is efficient and streamlined, thanks to Python's object-oriented features and robust third-party SDKs. With a modular design, this service can easily accommodate additional notification providers in the future.