Unleashing Python's Hidden Power: Profiling for Performance Optimization
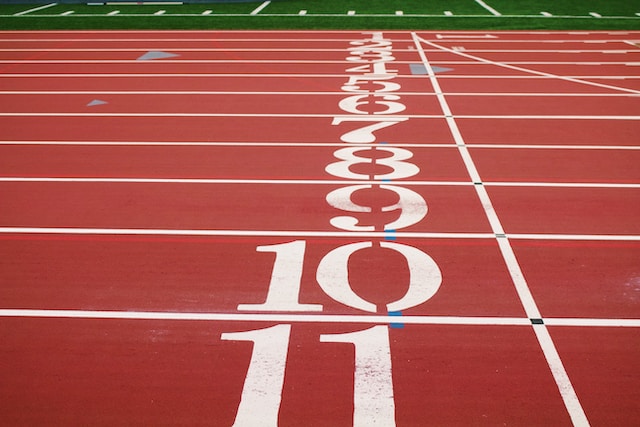
Profiling is a fundamental technique in software development that enables programmers to identify and rectify performance bottlenecks in their code. Python offers several built-in and third-party profiling tools to help developers optimize their applications. In this article, we'll delve into Python's standard library profiling modules, cProfile
and profile
, and introduce two popular third-party profilers: memory-profiler
and line-profiler
.
Understanding Profiling
Profiling involves measuring the execution time, memory usage, and other relevant metrics of a program to pinpoint areas that could be optimized. It aids developers in making informed decisions about code improvements, leading to enhanced performance and better resource utilization.
cProfile and profile: Python's Standard Profiling Modules
cProfile
cProfile
is a C-extension-based profiler included in Python's standard library. It provides detailed information about function calls, execution times, and call counts. Here's how you can use it:
import cProfile
def example_function():
total = 0
for i in range(1000):
total += i
return total
profiler = cProfile.Profile()
profiler.enable()
result = example_function()
profiler.disable()
profiler.print_stats()
profile
The profile module is a pure Python profiler that is less efficient than cProfile but is more portable:
import profile
def example_function():
total = 0
for i in range(1000):
total += i
return total
profiler = profile.Profile()
result = profiler.runcall(example_function)
profiler.print_stats()
Third-Party Profilers
memory-profiler
The memory-profiler
is a third-party profiler that focuses on memory usage. It helps identify memory-hungry parts of your code. Here's how you can use it:
- Install the package:
pip install memory-profiler
- Decorate the function you want to profile:
from memory_profiler import profile
@profile
def example_function():
total = 0
for i in range(1000):
total += i
return total
result = example_function()
- Run the script using the
mprof
command (requiresmatplotlib
):
pip install matplotlib # Install matplotlib if not already installed
mprof run script.py
mprof plot
line-profiler
The line-profiler
is another third-party profiler that provides insights into line-by-line execution times. It helps you identify specific lines causing performance bottlenecks. Here's how to use it:
- Install the package:
pip install line-profiler
- Decorate the function you want to profile:
from line_profiler import profile
@profile
def is_prime(n):
max_val = n ** 0.5
stop = int(max_val + 1)
for i in range(2, stop):
if n % i == 0:
return False
return True
@profile
def find_primes(size):
primes = []
for n in range(size):
flag = is_prime(n)
if flag:
primes.append(n)
return primes
@profile
def main():
print('start calculating')
primes = find_primes(100000)
print(f'done calculating. Found {len(primes)} primes.')
if __name__ == "__main__":
main()
Conclusion
Profiling is a critical step in the software development process that enables developers to optimize their code for better performance. Python provides cProfile and profile as built-in profilers, while third-party tools like memory-profiler and line-profiler offer additional insights into memory usage and line-by-line execution times.
By using these profiling tools effectively, developers can identify and address bottlenecks, resulting in faster, more efficient, and more responsive Python applications. Choose the appropriate profiler based on your project's requirements, and harness the power of profiling to deliver top-notch software.