Mastering the Adapter Design Pattern in Go
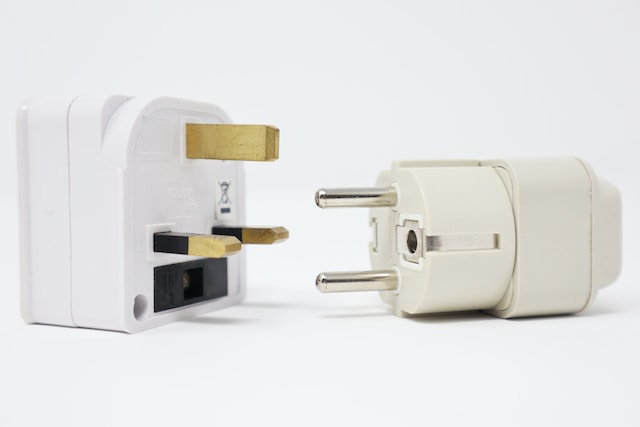
In the realm of software development, systems are often composed of diverse components, some of which have incompatible interfaces. This is where the Adapter design pattern comes to the rescue. The Adapter pattern enables objects with disparate interfaces to collaborate effectively, fostering code reusability, interoperability, and maintainability. In this article, we'll delve deep into the Adapter pattern, exploring its underlying principles, benefits, and showcasing advanced examples implemented in the Go programming language.
Understanding the Adapter Pattern
The Adapter pattern, categorized under structural design patterns, provides a solution for integrating systems that might otherwise be incompatible due to differences in interfaces. By creating a bridge between these components, the pattern ensures that they can interact seamlessly without the need for extensive modifications.
Benefits of Using the Adapter Pattern
-
Code Reusability: The Adapter pattern encourages reusing existing code and components without the need to rewrite or modify them extensively.
-
Interoperability: It facilitates the integration of third-party libraries or legacy codebases with modern systems, promoting collaboration.
-
Maintainability: The pattern enhances code cleanliness by centralizing adaptation logic, preventing codebase clutter and workarounds.
Implementing the Adapter Pattern in Go
Let's explore a more advanced example to illustrate the power of the Adapter pattern in Go. Imagine a scenario where a legacy XMLParser
needs to be adapted to work with a modern JSONParser
interface.
package main
import (
"fmt"
)
// Legacy XMLParser
type XMLParser struct{}
func (x *XMLParser) ParseXML(data string) {
fmt.Printf("Parsing XML: %s\n", data)
}
// Modern JSONParser interface
type JSONParser interface {
ParseJSON(data string)
}
// Adapter to make XMLParser work with JSONParser
type XMLJSONAdapter struct {
XMLParser *XMLParser
}
func (a *XMLJSONAdapter) ParseJSON(data string) {
adaptedData := "<converted>" + data + "</converted>"
a.XMLParser.ParseXML(adaptedData)
}
func main() {
xmlParser := &XMLParser{}
adapter := &XMLJSONAdapter{XMLParser: xmlParser}
data := `{"key": "value"}`
adapter.ParseJSON(data)
}
In this example, the XMLJSONAdapter
adapts the legacy XMLParser
to work with the modern JSONParser
interface, enabling the parsing of JSON data using the XML parser.
Real-World Use Case: Database Adapters
A common real-world scenario where the Adapter pattern shines is in database connectivity. Different databases often have unique APIs, making direct integration challenging. Adapters can bridge the gap, allowing a unified interface for working with diverse database systems.
Conclusion
The Adapter design pattern is a vital tool for seamlessly integrating components with disparate interfaces. By enabling effective collaboration between otherwise incompatible systems, the Adapter pattern promotes code reusability, interoperability, and maintainability. Whether adapting legacy code or integrating third-party libraries, the Adapter pattern plays a pivotal role in achieving efficient and organized software development. As you continue to master this pattern, you'll find yourself equipped with a powerful tool to conquer interface compatibility challenges in your software projects.