Exploring the Option Struct Pattern in Go
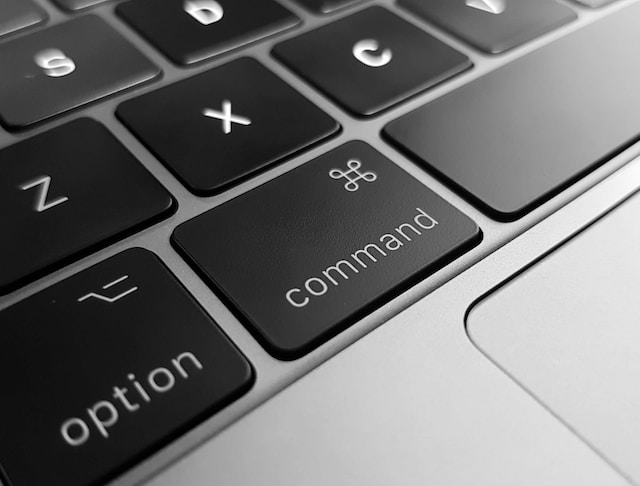
When working with the Go programming language, developers often encounter situations where functions may return values that could be missing or have a special meaning. The Option struct pattern is a powerful approach to address this, providing a clean and idiomatic way to handle optional values and errors. In this article, we'll delve into why and how Go uses the Option struct pattern.
The Problem of Optional Values and Errors
In Go, functions often return multiple values, where one of them represents an error. However, some functions may also return optional values that indicate the absence of data or a specific condition. This can make code harder to read and maintain, as developers must constantly check for errors and optional values, leading to code clutter.
Introducing the Option Struct
The Option struct pattern is a design approach that uses a custom type, usually called Option
or a similar name, to wrap values that could be absent. This pattern enhances code readability by making the presence or absence of a value explicit, removing the need for constant error checks.
Here's an example of how the Option struct pattern works in Go:
package main
import (
"errors"
"fmt"
)
// Option represents an optional value or an error
type Option struct {
Value interface{}
Err error
}
// NewOption creates a new Option with a value
func NewOption(val interface{}) Option {
return Option{Value: val}
}
// NewErrorOption creates a new Option with an error
func NewErrorOption(err error) Option {
return Option{Err: err}
}
// main function demonstrates the use of Option struct
func main() {
data := []int{1, 2, 3, 4, 5}
result := findElement(data, 6)
if result.Err != nil {
fmt.Println("Error:", result.Err)
} else {
fmt.Println("Value found:", result.Value)
}
}
// findElement searches for an element in a slice
func findElement(data []int, target int) Option {
for _, val := range data {
if val == target {
return NewOption(val)
}
}
return NewErrorOption(errors.New("Element not found"))
}
In this example, the Option struct wraps the value or error, making the code more self-explanatory. Functions like NewOption and NewErrorOption help create instances of the Option struct with the appropriate value or error.
Benefits of the Option Struct Pattern
- Improved Readability: The Option struct makes it clear whether a value is present or not, reducing the need for complex error handling logic.
- Simpler Error Handling: By encapsulating the error within the Option struct, you can handle errors uniformly without mixing them with normal values.
- Clearer Intent: Code that uses the Option struct pattern conveys the intention explicitly, making the developer's intent clearer and reducing ambiguity.
Conclusion
The Option struct pattern is a valuable technique in Go for handling optional values and errors. By encapsulating values and errors within a single construct, Go developers can write more concise, readable, and maintainable code. This pattern aligns with Go's philosophy of simplicity and clarity, making it a powerful tool for writing clean and effective programs.