Implementing the Singleton Design Pattern in Go
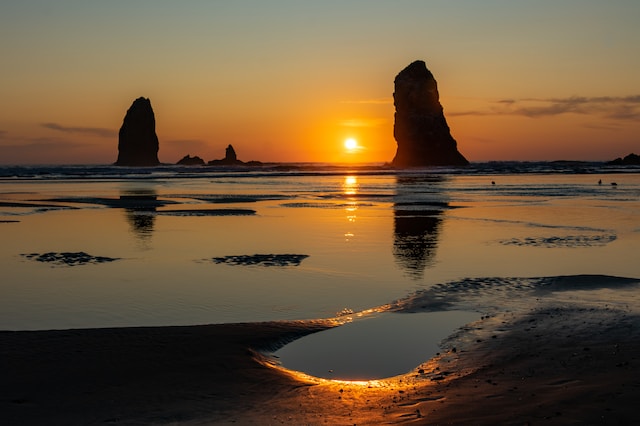
In software design, the Singleton pattern is a creational design pattern that ensures a class has only one instance and provides a global point of access to that instance. In this article, we'll explore how to implement the Singleton pattern in the Go programming language for a Pelican Markdown article website.
The Singleton Design Pattern
The Singleton pattern is particularly useful when you want to ensure there's only one instance of a class, such as a configuration manager or a database connection pool. This can prevent resource wastage and synchronization issues.
Implementing Singleton in Go
Let's implement a Singleton pattern for managing Pelican Markdown articles in Go.
package main
import (
"sync"
)
// Article represents a Pelican Markdown article
type Article struct {
Title string
Content string
}
// SingletonArticleManager is a struct that manages a single instance of Article
type SingletonArticleManager struct {
article *Article
once sync.Once
}
var instance *SingletonArticleManager
// GetInstance returns the single instance of SingletonArticleManager
func GetInstance() *SingletonArticleManager {
if instance == nil {
instance = &SingletonArticleManager{}
instance.init()
}
return instance
}
// init is used to initialize the singleton instance
func (s *SingletonArticleManager) init() {
s.once.Do(func() {
// Initialize the default article
s.article = &Article{
Title: "Default Title",
Content: "Default Content",
}
})
}
// GetArticle returns the current Article managed by the singleton
func (s *SingletonArticleManager) GetArticle() *Article {
return s.article
}
// UpdateArticle updates the content of the managed Article
func (s *SingletonArticleManager) UpdateArticle(title, content string) {
s.article.Title = title
s.article.Content = content
}
func main() {
// Usage example
manager := GetInstance()
// Get the managed article
article := manager.GetArticle()
// Print article details
println("Article Title:", article.Title)
println("Article Content:", article.Content)
}
In this example, the SingletonArticleManager
struct manages a single instance of the Article struct. We use the sync.Once type to ensure that initialization happens only once. The GetInstance function provides access to the singleton instance.
Conclusion
The Singleton design pattern is a powerful tool for ensuring a single instance of a class in your application. In Go, you can implement this pattern using synchronization mechanisms like sync.Once to ensure safe and efficient initialization.