Integrating pprof with Gin
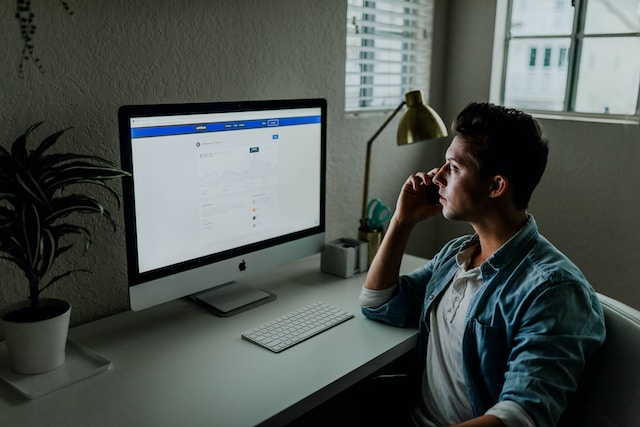
Profiling applications is crucial for understanding performance bottlenecks. In Go, the standard library offers the pprof
package for this purpose. This article covers how to integrate pprof
with the Gin web framework using the gin-contrib/pprof
package.
Prerequisites
- A Go development environment.
- Knowledge of the Gin framework.
Setting Up Gin
Install Gin:
go get -u github.com/gin-gonic/gin
Create a simple Gin
application:
package main
import "github.com/gin-gonic/gin"
func main() {
r := gin.Default()
r.GET("/", func(c *gin.Context) {
c.String(200, "Welcome to Gin!")
})
r.Run(":8080")
}
If you run the application and visit http://localhost:8080/
, you should see "Welcome to Gin!".
Integrating pprof
using gin-contrib/pprof
- Start by installing the
gin-contrib/pprof
package:
go get -u github.com/gin-contrib/pprof
- Modify your Gin application to integrate
pprof
:
package main
import (
"github.com/gin-gonic/gin"
"github.com/gin-contrib/pprof"
)
func main() {
r := gin.Default()
r.GET("/", func(c *gin.Context) {
c.String(200, "Welcome to Gin!")
})
// Use the pprof middleware
pprof.Register(r)
r.Run(":8080")
}
The pprof.Register(r)
function attaches all standard net/http/pprof
handlers to the provided *gin.Engine
.
Accessing Profiling Data
After starting your application:
- Visit
http://localhost:8080/debug/pprof/
for an overview. - Navigate to
http://localhost:8080/debug/pprof/heap
for heap profile data. - Use
http://localhost:8080/debug/pprof/goroutine
for a goroutine profile.
... and so on for other available profiles.
Conclusion
Using gin-contrib/pprof
simplifies the process of integrating Go's built-in pprof
profiling tools with the Gin framework. By taking advantage of this integration, developers can quickly gather performance data on their web applications, making it easier to optimize and debug. Happy profiling!