A Comprehensive Dive into `go-jet`
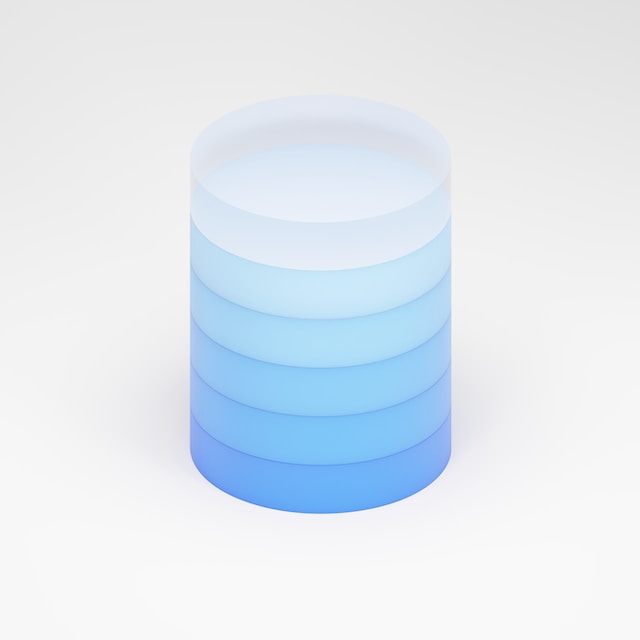
When it comes to working with databases in Go, several packages promise efficiency and flexibility. Yet, not many combine the raw prowess of SQL with Go's type-safe environment. Enter go-jet
.
What Sets go-jet
Apart?
Most Go developers face a conundrum: The convenience of ORMs or the power of raw SQL? go-jet
doesn't make you choose. It integrates the best of both worlds:
-
Type Safety: No more runtime SQL errors. SQL errors, a common pain point, get caught during compile-time, ensuring robust applications.
-
Expressiveness: The syntax, being highly expressive, doesn't feel alien to Go developers. This means writing SQL that's readable, intuitive, and Go-ish.
-
Performance: Abstractions usually come at a cost, but
go-jet
strives to minimize overheads, ensuring applications run efficiently.
Deep Dive into go-jet
Syntax and Features
go-jet
has a treasure trove of features and functionalities. Here's a breakdown:
SELECT Queries
go-jet
excels at making SELECT queries intuitive:
var users []Users
err = Users.SELECT(Users.AllColumns).FROM(Users).WHERE(Users.Age.GT(Int(18))).Query(db, &users)
Notice the chaining and the usage of methods like GT
(Greater Than). This makes the code readable, yet strictly type-checked.
INSERT, UPDATE, and DELETE
DML operations are straightforward and flexible:
// Insert
insertStmt := Users.INSERT(Users.Name, Users.Age).VALUES("Alice", 28)
_, err = insertStmt.Exec(db)
// Update
updateStmt := Users.UPDATE().SET(Users.Name.SET(String("Bob"))).WHERE(Users.ID.EQ(Int(1)))
_, err = updateStmt.Exec(db)
// Delete
deleteStmt := Users.DELETE().WHERE(Users.Age.LT(Int(18)))
_, err = deleteStmt.Exec(db)
Joins, Aggregations, and More
go-jet
is not limited to simple CRUD operations:
// Join operations
joinQuery := Orders.
INNER_JOIN(Customers, Orders.CustomerID.EQ(Customers.ID)).
SELECT(Orders.AllColumns, Customers.AllColumns).
WHERE(Customers.Country.EQ(String("USA")))
// Aggregation
countQuery := Orders.
SELECT(Orders.CustomerID, COUNT(Orders.ID).AS("TotalOrders")).
GROUP_BY(Orders.CustomerID)
// Complex conditions
complexCondition := Orders.ShippedDate.IS_NOT_NULL().AND(Orders.Freight.GT(Float(50)))
These examples merely scratch the surface. With subqueries, UNION operations, transactions, and more, go-jet
offers a comprehensive toolkit for database operations in Go.
Real-world Usage: When Should You Opt for go-jet
?
The value of any library is its applicability in real-world scenarios. For go-jet
:
-
Large-scale applications: With increasing complexity, type-safety becomes invaluable.
go-jet
minimizes runtime errors, ensuring large-scale applications remain robust. -
Migration from another ORM: If you're coming from a less type-safe environment and want to leverage Go's type safety,
go-jet
serves as an excellent bridge. -
When raw SQL and ORM need to coexist: Not all queries fit the ORM mold.
go-jet
provides the flexibility of raw SQL alongside ORM-style operations.
Concluding Thoughts
Databases lie at the heart of most applications. While Go offers built-in tools for database operations, go-jet
augments this experience. It balances raw SQL's power with ORM convenience, all wrapped up in a type-safe environment. As Go's ecosystem continues to flourish, tools like go-jet
ensure that database operations remain streamlined and efficient.